First Steps with Barcode Reader SDK for .NET
Barcode Reader SDK for .NET can be used in Visual Studio IDE for adding barcode recognition capabilities to your .NET Applications. You can use the Barcode Reader SDK to scan a digital image looking for the desired barcode symbology and decode it (meaning to get the data encoded by the barcode) inside your .NET application.
A simple sample code for barcode recognition
Suppose you need to decode the barcode symbols shown in the following image:
This image contains an EAN-13 and a Code-128 barcodes. The following code will scan this image file looking for the barcodes and then shows the result in the Console window.
1. Open Visual Studio and create a new Console Application using your preferred .NET language.
2. Add a reference to System.Drawing.dll and Neodynamic.SDK.BarcodeReader.dll
3. Copy the following code in the Console's main method:
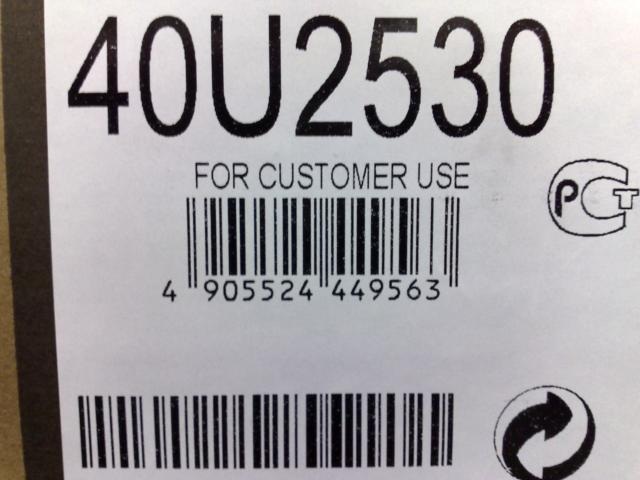
This image contains an EAN-13 and a Code-128 barcodes. The following code will scan this image file looking for the barcodes and then shows the result in the Console window.
1. Open Visual Studio and create a new Console Application using your preferred .NET language.
2. Add a reference to System.Drawing.dll and Neodynamic.SDK.BarcodeReader.dll
3. Copy the following code in the Console's main method:
VB.NET
'Add the Barcode Reader namespace reference Imports Neodynamic.SDK.BarcodeReader
'Create a BarcodeReader object Dim myReader As New BarcodeReader() 'Add the barcode symbologies to be recognized myReader.Symbology.Add(Symbology.Ean13) myReader.Symbology.Add(Symbology.Code128) 'Set max num of barcode symbols to be detected myReader.Hints.MaxNumOfBarcodes = 2 'Scan the source image and get result Dim results As List(Of BarcodeScanResult) = myReader.Scan("c:\barcode_sample.jpg") 'Display scan result For Each result As BarcodeScanResult In results Console.WriteLine("Barcode Type: " & result.Symbology.ToString()) Console.WriteLine("Barcode Data: " + result.Text) Console.WriteLine("=============") Next
C#
//Add the Barcode Reader namespace reference using Neodynamic.SDK.BarcodeReader;
//Create a BarcodeReader object BarcodeReader myReader = new BarcodeReader(); //Add the barcode symbologies to be recognized myReader.Symbology.Add(Symbology.Ean13); myReader.Symbology.Add(Symbology.Code128); //Set max num of barcode symbols to be detected myReader.Hints.MaxNumOfBarcodes = 2; //Scan the source image and get result List<BarcodeScanResult> results = myReader.Scan(@"c:\barcode_sample.jpg"); //Display scan result foreach (BarcodeScanResult result in results) { Console.WriteLine("Barcode Type: " + result.Symbology.ToString()); Console.WriteLine("Barcode Data: " + result.Text); Console.WriteLine("============="); }
Barcode Reader App Sample
We've developed a more complete Barcode Reader application which you can examine for more advanced settings. The sample app is provided with source code in C# and VB and you can find them in the installation folder of Barcode Reader SDK.