Shape Items (Lines, Rectangles, Circles, Ellipses, Tables/Grids)
ThermalLabel SDK supports AutoShapes which are preset shapes like rectangles, circles, lines, ellipses, and Tables/Grids that can be placed on the label surface.
- In This Section
- Rectangle Item
- Line Item
- Circle and Ellipse Items
- Table/Grid Item
Rectangle Item
A Rectangle Item – represented by Neodynamic.SDK.Printing.RectangleShapeItem class – lets you draw rectangle shapes on the label. RectangleShapeItem objects are created by specifying some basic properties such as Width, Height, Fill, StrokeFill, Roundness, etc.
RectangleShapeItem Example:
By using RectangleShapeItem objects you can draw rectangles as well as rounded rectangles. The following figure is a sample label featuring rectangle shapes.
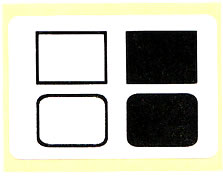
The following are the VB.NET and C# codes for generating that basic label featuring rectangles and rounded rectangles.
NOTE
In EPL-based printers roundness is not supported.
In EPL-based printers roundness is not supported.
RectangleShapeItem Example:
By using RectangleShapeItem objects you can draw rectangles as well as rounded rectangles. The following figure is a sample label featuring rectangle shapes.
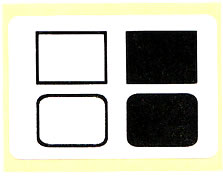
NOTE
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following are the VB.NET and C# codes for generating that basic label featuring rectangles and rounded rectangles.
NOTE: Please be aware that in the following code we are using Centimeter as the Unit of Measurement.
Visual Basic
'Define a ThermalLabel object and set unit to cm and label size Dim tLabel As New ThermalLabel(UnitType.Cm, 6, 0) 'Define some RectangleShapeItem objects Dim r1 As New RectangleShapeItem(0.75, 0.5, 2, 1.5) 'Set stroke thickness r1.StrokeThickness = 0.1 Dim r2 As New RectangleShapeItem(0.75, 2.25, 2, 1.5) 'Set stroke thickness r2.StrokeThickness = 0.1 'Set roundness r2.Roundness = 0.25 Dim r3 As New RectangleShapeItem(3.25, 0.5, 2, 1.5) 'Set fill color... r3.FillColor = Color.Black Dim r4 As New RectangleShapeItem(3.25, 2.25, 2, 1.5) 'Set roundness r4.Roundness = 0.25 'Set fill color... r4.FillColor = Color.Black 'Add items to ThermalLabel object... tLabel.Items.Add(r1) tLabel.Items.Add(r2) tLabel.Items.Add(r3) tLabel.Items.Add(r4) 'Create a PrintJob object Dim pj As New PrintJob() 'Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB 'Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203 'Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL 'Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z" 'Print ThermalLabel object... pj.Print(tLabel)
C#
//Define a ThermalLabel object and set unit to cm and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Cm, 6, 0); //Define some RectangleShapeItem objects RectangleShapeItem r1 = new RectangleShapeItem(0.75, 0.5, 2, 1.5); //Set stroke thickness r1.StrokeThickness = 0.1; RectangleShapeItem r2 = new RectangleShapeItem(0.75, 2.25, 2, 1.5); //Set stroke thickness r2.StrokeThickness = 0.1; //Set roundness r2.Roundness = 0.25; RectangleShapeItem r3 = new RectangleShapeItem(3.25, 0.5, 2, 1.5); //Set fill color... r3.FillColor = Color.Black; RectangleShapeItem r4 = new RectangleShapeItem(3.25, 2.25, 2, 1.5); //Set roundness r4.Roundness = 0.25; //Set fill color... r4.FillColor = Color.Black; //Add items to ThermalLabel object... tLabel.Items.Add(r1); tLabel.Items.Add(r2); tLabel.Items.Add(r3); tLabel.Items.Add(r4); //Create a PrintJob object PrintJob pj = new PrintJob(); //Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB; //Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203; //Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL; //Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z"; //Print ThermalLabel object... pj.Print(tLabel);
Line Item
A Line Item – represented by Neodynamic.SDK.Printing.LineShapeItem class – lets you draw lines on the label. LineShapeItem objects are created by specifying some basic properties such as Width, Height, Orientation, etc.
LineShapeItem Example:
By using LineShapeItem objects you can draw lines at any orientation i.e. horizontal, vertical, diagonals up and down. The following figure is a sample label featuring line shapes.
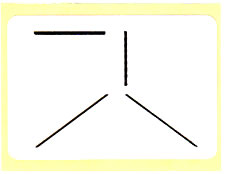
The following are the VB.NET and C# codes for generating that basic label featuring lines.
LineShapeItem Example:
By using LineShapeItem objects you can draw lines at any orientation i.e. horizontal, vertical, diagonals up and down. The following figure is a sample label featuring line shapes.
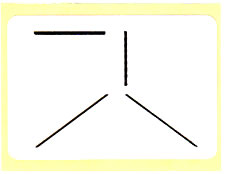
NOTE
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following are the VB.NET and C# codes for generating that basic label featuring lines.
NOTE: Please be aware that in the following code we are using Centimeter as the Unit of Measurement.
Visual Basic
'Define a ThermalLabel object and set unit to cm and label size Dim tLabel As New ThermalLabel(UnitType.Cm, 6, 0) 'Define some LineShapeItem objects Dim l1 As New LineShapeItem(0.75, 0.5, 2, 1.5) 'Set stroke thickness l1.StrokeThickness = 0.1 'Set orientation l1.Orientation = LineOrientation.Horizontal Dim l2 As New LineShapeItem(0.75, 2.25, 2, 1.5) 'Set stroke thickness l2.StrokeThickness = 0.1 'Set orientation l2.Orientation = LineOrientation.DiagonalUp Dim l3 As New LineShapeItem(3.25, 0.5, 2, 1.5) 'Set stroke thickness l3.StrokeThickness = 0.1 'Set orientation l3.Orientation = LineOrientation.Vertical Dim l4 As New LineShapeItem(3.25, 2.25, 2, 1.5) 'Set stroke thickness l4.StrokeThickness = 0.1 'Set orientation l4.Orientation = LineOrientation.DiagonalDown 'Add items to ThermalLabel object... tLabel.Items.Add(l1) tLabel.Items.Add(l2) tLabel.Items.Add(l3) tLabel.Items.Add(l4) 'Create a PrintJob object Dim pj As New PrintJob() 'Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB 'Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203 'Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL 'Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z" 'Print ThermalLabel object... pj.Print(tLabel)
C#
//Define a ThermalLabel object and set unit to cm and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Cm, 6, 0); //Define some LineShapeItem objects LineShapeItem l1 = new LineShapeItem(0.75, 0.5, 2, 1.5); //Set stroke thickness l1.StrokeThickness = 0.1; //Set orientation l1.Orientation = LineOrientation.Horizontal; LineShapeItem l2 = new LineShapeItem(0.75, 2.25, 2, 1.5); //Set stroke thickness l2.StrokeThickness = 0.1; //Set orientation l2.Orientation = LineOrientation.DiagonalUp; LineShapeItem l3 = new LineShapeItem(3.25, 0.5, 2, 1.5); //Set stroke thickness l3.StrokeThickness = 0.1; //Set orientation l3.Orientation = LineOrientation.Vertical; LineShapeItem l4 = new LineShapeItem(3.25, 2.25, 2, 1.5); //Set stroke thickness l4.StrokeThickness = 0.1; //Set orientation l4.Orientation = LineOrientation.DiagonalDown; //Add items to ThermalLabel object... tLabel.Items.Add(l1); tLabel.Items.Add(l2); tLabel.Items.Add(l3); tLabel.Items.Add(l4); //Create a PrintJob object PrintJob pj = new PrintJob(); //Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB; //Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203; //Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL; //Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z"; //Print ThermalLabel object... pj.Print(tLabel);
Circle and Ellipse Items
Circle and Ellipse items – represented by Neodynamic.SDK.Printing.CircleShapeItem and Neodynamic.SDK.Printing.EllipseShapeItem classes respectively– let you draw circle and ellipse/oval shapes on the label. Both kinds of objects are created by specifying some basic properties such as Width, Height, Fill, StrokeFill, etc.
CircleShapeItem and EllipseShapeItem Example:
By using CircleShapeItem and EllipseShapeItem objects you can draw circle, ellipse and oval shapes. The following figure is a sample label featuring such shapes.
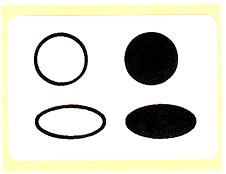
The following are the VB.NET and C# codes for generating that basic label featuring circles and ellipses.
CircleShapeItem and EllipseShapeItem Example:
By using CircleShapeItem and EllipseShapeItem objects you can draw circle, ellipse and oval shapes. The following figure is a sample label featuring such shapes.
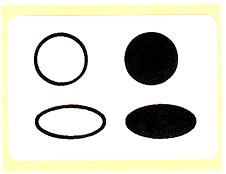
NOTE
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following are the VB.NET and C# codes for generating that basic label featuring circles and ellipses.
NOTE: Please be aware that in the following code we are using Centimeter as the Unit of Measurement.
Visual Basic
'Define a ThermalLabel object and set unit to cm and label size Dim tLabel As New ThermalLabel(UnitType.Cm, 6, 0) 'Define some CircleShapeItem objects Dim c1 As New CircleShapeItem(0.75, 0.5, 1.5) 'Set stroke thickness c1.StrokeThickness = 0.1 Dim c2 As New CircleShapeItem(3.25, 0.5, 1.5) 'Set fill color... c2.FillColor = Color.Black 'Define some EllipseShapeItem objects Dim e1 As New EllipseShapeItem(0.75, 2.5, 2, 1) 'Set stroke thickness e1.StrokeThickness = 0.1 Dim e2 As New EllipseShapeItem(3.25, 2.5, 2, 1) 'Set fill color... e2.FillColor = Color.Black 'Add items to ThermalLabel object... tLabel.Items.Add(c1) tLabel.Items.Add(c2) tLabel.Items.Add(e1) tLabel.Items.Add(e2) 'Create a PrintJob object Dim pj As New PrintJob() 'Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB 'Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203 'Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL 'Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z" 'Print ThermalLabel object... pj.Print(tLabel)
C#
//Define a ThermalLabel object and set unit to cm and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Cm, 6, 0); //Define some CircleShapeItem objects CircleShapeItem c1 = new CircleShapeItem(0.75, 0.5, 1.5); //Set stroke thickness c1.StrokeThickness = 0.1; CircleShapeItem c2 = new CircleShapeItem(3.25, 0.5, 1.5); //Set fill color... c2.FillColor = Color.Black; //Define some EllipseShapeItem objects EllipseShapeItem e1 = new EllipseShapeItem(0.75, 2.5, 2, 1); //Set stroke thickness e1.StrokeThickness = 0.1; EllipseShapeItem e2 = new EllipseShapeItem(3.25, 2.5, 2, 1); //Set fill color... e2.FillColor = Color.Black; //Add items to ThermalLabel object... tLabel.Items.Add(c1); tLabel.Items.Add(c2); tLabel.Items.Add(e1); tLabel.Items.Add(e2); //Create a PrintJob object PrintJob pj = new PrintJob(); //Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB; //Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203; //Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL; //Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z"; //Print ThermalLabel object... pj.Print(tLabel);
Table/Grid Item
A Table Item – represented by Neodynamic.SDK.Printing.TableShapeItem class – lets you draw table and grid layouts on the label. TableShapeItem objects are created by specifying some basic properties such as Width, Height, number of Columns & Rows, etc.
TableShapeItem objects are very flexible allowing you to create uniform grids and supporting column/row span as well as cell spacing and roundness.
TableShapeItem Examples:
Example #1: The following figure is a sample label featuring a Table composed of 2 columns and 3 rows.
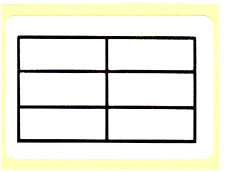
The following is the VB.NET and C# codes for generating that basic label featuring a table composed of 2 columns and 3 rows.
Example #2: The following figure is a sample label featuring a Table composed of 2 columns and 3 rows. The table features a cell spacing setting and the first cell (row = 0, col = 0) has column span 2 as well as a text.
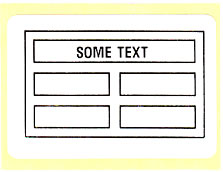
The following is the VB.NET and C# codes for generating that basic label featuring the involved table.
Example #3: The following figure is a sample label featuring a Table composed of 1 column and 2 rows. The table features a cell spacing setting and cells roundness. The first cell (row = 0, col = 0) has a text and the second cell (row = 1, col = 0) has a barcode.
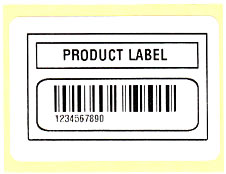
The following is the VB.NET and C# codes for generating that basic label featuring the involved table.
TableShapeItem objects are very flexible allowing you to create uniform grids and supporting column/row span as well as cell spacing and roundness.
NOTE
In EPL-based printers roundness is not supported.
In EPL-based printers roundness is not supported.
TableShapeItem Examples:
Example #1: The following figure is a sample label featuring a Table composed of 2 columns and 3 rows.
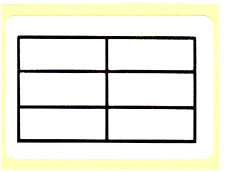
NOTE
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following is the VB.NET and C# codes for generating that basic label featuring a table composed of 2 columns and 3 rows.
NOTE: Please be aware that in the following code we are using Centimeter as the Unit of Measurement.
Visual Basic
'Define a ThermalLabel object and set unit to cm and label size Dim tLabel As New ThermalLabel(UnitType.Cm, 6, 0) 'Define a TableShapeItem object Dim table As New TableShapeItem(0.5, 0.5, 5, 3, 2, 3) 'Set stroke thickness table.StrokeThickness = 0.1 'Add items to ThermalLabel object... tLabel.Items.Add(table) 'Create a PrintJob object Dim pj As New PrintJob() 'Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB 'Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203 'Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL 'Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z" 'Print ThermalLabel object... pj.Print(tLabel)
C#
//Define a ThermalLabel object and set unit to cm and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Cm, 6, 0); //Define a TableShapeItem object TableShapeItem table = new TableShapeItem(0.5, 0.5, 5, 3, 2, 3); //Set stroke thickness table.StrokeThickness = 0.1; //Add items to ThermalLabel object... tLabel.Items.Add(table); //Create a PrintJob object PrintJob pj = new PrintJob(); //Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB; //Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203; //Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL; //Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z"; //Print ThermalLabel object... pj.Print(tLabel);
Example #2: The following figure is a sample label featuring a Table composed of 2 columns and 3 rows. The table features a cell spacing setting and the first cell (row = 0, col = 0) has column span 2 as well as a text.
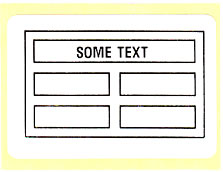
NOTE
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following is the VB.NET and C# codes for generating that basic label featuring the involved table.
NOTE: Please be aware that in the following code we are using Centimeter as the Unit of Measurement.
Visual Basic
'Define a ThermalLabel object and set unit to cm and label size Dim tLabel As New ThermalLabel(UnitType.Cm, 6, 0) 'Define a TableShapeItem object Dim table As New TableShapeItem(0.5, 0.5, 5, 3, 2, 3) 'Set stroke thickness table.StrokeThickness = 0.05 'Set cell spacing table.CellSpacing = 0.25 'Cell settings... table.Cells(0, 0).ColSpan = 2 table.Cells(0, 0).Padding.Top = 0.2 table.Cells(0, 0).Content = CellContent.Text table.Cells(0, 0).ContentText.Text = "SOME TEXT" table.Cells(0, 0).ContentText.TextAlignment = TextAlignment.Center table.Cells(0, 0).ContentText.Font.CharHeight = 12 'Add items to ThermalLabel object... tLabel.Items.Add(table) 'Create a PrintJob object Dim pj As New PrintJob() 'Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB 'Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203 'Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL 'Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z" 'Print ThermalLabel object... pj.Print(tLabel)
C#
//Define a ThermalLabel object and set unit to cm and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Cm, 6, 0); //Define a TableShapeItem object TableShapeItem table = new TableShapeItem(0.5, 0.5, 5, 3, 2, 3); //Set stroke thickness table.StrokeThickness = 0.05; //Set cell spacing table.CellSpacing = 0.25; //Cell settings... table.Cells[0, 0].ColSpan = 2; table.Cells[0, 0].Padding.Top = 0.2; table.Cells[0, 0].Content = CellContent.Text; table.Cells[0, 0].ContentText.Text = "SOME TEXT"; table.Cells[0, 0].ContentText.TextAlignment = TextAlignment.Center; table.Cells[0, 0].ContentText.Font.CharHeight = 12; //Add items to ThermalLabel object... tLabel.Items.Add(table); //Create a PrintJob object PrintJob pj = new PrintJob(); //Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB; //Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203; //Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL; //Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z"; //Print ThermalLabel object... pj.Print(tLabel);
Example #3: The following figure is a sample label featuring a Table composed of 1 column and 2 rows. The table features a cell spacing setting and cells roundness. The first cell (row = 0, col = 0) has a text and the second cell (row = 1, col = 0) has a barcode.
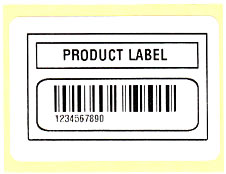
NOTE
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following output is from a ZPL printer. Other printers based on different programming languages like EPL will produce different outputs.
The following is the VB.NET and C# codes for generating that basic label featuring the involved table.
NOTE: Please be aware that in the following code we are using Centimeter as the Unit of Measurement.
Visual Basic
'Define a ThermalLabel object and set unit to cm and label size Dim tLabel As New ThermalLabel(UnitType.Cm, 6, 0) 'Define a TableShapeItem object Dim table As New TableShapeItem(0.5, 0.5, 5, 3, 1, 2) 'Set stroke thickness table.StrokeThickness = 0.05 'Set cell spacing table.CellSpacing = 0.25 'Set first row height table.Rows(0).Height = 0.75 'First Cell settings... table.Cells(0, 0).Padding.Top = 0.2 table.Cells(0, 0).Content = CellContent.Text table.Cells(0, 0).ContentText.Text = "PRODUCT LABEL" table.Cells(0, 0).ContentText.TextAlignment = TextAlignment.Center table.Cells(0, 0).ContentText.Font.CharHeight = 12 'Second Cell settings... table.Cells(1, 0).Roundness = 0.25 table.Cells(1, 0).Padding.Top = 0.2 table.Cells(1, 0).Padding.Left = 0.5 table.Cells(1, 0).Content = CellContent.Barcode table.Cells(1, 0).ContentBarcode.Symbology = BarcodeSymbology.Code128 table.Cells(1, 0).ContentBarcode.Code = "1234567890" table.Cells(1, 0).ContentBarcode.BarWidth = 0.04 table.Cells(1, 0).ContentBarcode.BarHeight = 0.75 'Add items to ThermalLabel object... tLabel.Items.Add(table) 'Create a PrintJob object Dim pj As New PrintJob() 'Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB 'Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203 'Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL 'Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z" 'Print ThermalLabel object... pj.Print(tLabel)
C#
//Define a ThermalLabel object and set unit to cm and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Cm, 6, 0); //Define a TableShapeItem object TableShapeItem table = new TableShapeItem(0.5, 0.5, 5, 3, 1, 2); //Set stroke thickness table.StrokeThickness = 0.05; //Set cell spacing table.CellSpacing = 0.25; //Set first row height table.Rows[0].Height = 0.75; //First Cell settings... table.Cells[0, 0].Padding.Top = 0.2; table.Cells[0, 0].Content = CellContent.Text; table.Cells[0, 0].ContentText.Text = "PRODUCT LABEL"; table.Cells[0, 0].ContentText.TextAlignment = TextAlignment.Center; table.Cells[0, 0].ContentText.Font.CharHeight = 12; //Second Cell settings... table.Cells[1, 0].Roundness = 0.25; table.Cells[1, 0].Padding.Top = 0.2; table.Cells[1, 0].Padding.Left = 0.5; table.Cells[1, 0].Content = CellContent.Barcode; table.Cells[1, 0].ContentBarcode.Symbology = BarcodeSymbology.Code128; table.Cells[1, 0].ContentBarcode.Code = "1234567890"; table.Cells[1, 0].ContentBarcode.BarWidth = 0.04; table.Cells[1, 0].ContentBarcode.BarHeight = 0.75; //Add items to ThermalLabel object... tLabel.Items.Add(table); //Create a PrintJob object PrintJob pj = new PrintJob(); //Thermal Printer is connected through USB pj.PrinterSettings.Communication.CommunicationType = CommunicationType.USB; //Set Thermal Printer resolution pj.PrinterSettings.Dpi = 203; //Set Thermal Printer language pj.PrinterSettings.ProgrammingLanguage = ProgrammingLanguage.ZPL; //Set Thermal Printer name pj.PrinterSettings.PrinterName = "Zebra TLP2844-Z"; //Print ThermalLabel object... pj.Print(tLabel);