In this section
Image, Graphic and Picture Items Overview
The ImageItem object
An ImageItem – represented by Neodynamic.SDK.Printing.ImageItem class – simply wraps an image, graphic or picture that must be drawn on the label. ImageItem objects are created by specifying some basic properties such as X, Y, Width, Height, Image source, etc.
The ImageItem object can wrap and manage the following image formats: BMP, GIF, JPEG, and PNG. The image content can be specified as a File path or URL, a base64 string, or a byte array. Once an image source is specified, you can control how to size, rotate, and flip it, as well as monochrome settings conversion supporting Threshold and Floyd-Steinberg algorithms and reversing.
After creating an ImageItem object, it will printed on the label if you add it to the Items collection property of the ThermalLabel object.
The ImageItem object can wrap and manage the following image formats: BMP, GIF, JPEG, and PNG. The image content can be specified as a File path or URL, a base64 string, or a byte array. Once an image source is specified, you can control how to size, rotate, and flip it, as well as monochrome settings conversion supporting Threshold and Floyd-Steinberg algorithms and reversing.
After creating an ImageItem object, it will printed on the label if you add it to the Items collection property of the ThermalLabel object.
Image Resizing
When you create an ImageItem, you must specify the source image as well as its location inside the label (through X & Y properties) and its printed size. The printed size of the image is configured by using the Width, Height and LockAspectRatio properties as follows:
- If LockAspectRatio = None, then the printed size of the image will be the one specified to Width & Height properties
- If LockAspectRatio = WidthBased, then the printed size of the image will be the one specified to Width property and the Height will be automatically calculated maintaining the original proportions.
- If LockAspectRatio = HeightBased, then the printed size of the image will be the one specified to Height property and the Width will be automatically calculated maintaining the original proportions.
- If LockAspectRatio = Fit, then the printed size of the image will be the one specified to Width & Height properties maintaining the original proportions.
Image Flipping and Rotation
You can specify that the source image be flipped when printing. The flipping is configured by using the Flip property allowing you to flip the source image horizontally, vertically or both directions.
You can also rotate the source image if needed. The rotation can be done at any angle (in degrees) by specifying it to the RotationAngle property. The rotation angle range from 0 to 360 degrees measured clockwise from the x-axis.
Internally, the image processing actions are performed in the following order: Resize > Flip > Rotate
You can also rotate the source image if needed. The rotation can be done at any angle (in degrees) by specifying it to the RotationAngle property. The rotation angle range from 0 to 360 degrees measured clockwise from the x-axis.
Internally, the image processing actions are performed in the following order: Resize > Flip > Rotate
Monochrome conversion
When printing an image to a thermal printer, the source image must be converted to monochrome format i.e. black & white colors. It is important you work with images in such basic color scheme when specifying the source image in order to avoid losing quality. Anyway, ImageItem are processed to monochrome by using the MonochromeSettings property.
ThermalLable SDK provides four Dithering Methods for black & white conversion which are set up on the DitherMethod property of MonochromeSettings object:
The MonochromeSettings property also allows you to create a "reverse" effect which means that the image shape will be printed as White On Black i.e. reversing the monochrome pixel conversion.
ThermalLable SDK provides four Dithering Methods for black & white conversion which are set up on the DitherMethod property of MonochromeSettings object:
- Threshold: it is the simplest dithering method which any pixel value below the threshold becomes black and any pixel value above the threshold becomes white. It is useful for simple images like logos or symbols.
- PatternDiffusion: this method uses a 4x4 dithering matrix to create a grouping of pixels that approximates the pixel group brightness. Use it for photos and other complicated images.
- FloydSteinberg: it uses Floyd-Steinberg algorithm to keep a track of the error in brightness caused by the threshold, and diffusing it across an area. Use it for photos and other complicated images.
- OtsuThreshold: it uses Otsu's method to automatically perform histogram shape-based image thresholding. Use it for simple images like logos or symbols.
The MonochromeSettings property also allows you to create a "reverse" effect which means that the image shape will be printed as White On Black i.e. reversing the monochrome pixel conversion.
ImageItem samples
Example #1: Below is a sample logo in JPEG format. The figure under the logo image is a sample label featuring such image but printed as monochrome by using an ImageItem object.
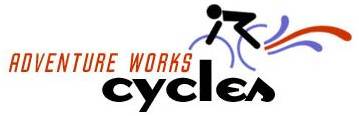
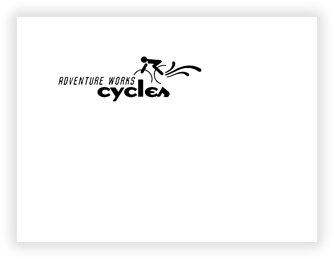
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 4, 3) tLabel.GapLength = 0.2 'Define an ImageItem object Dim logo As New ImageItem(0.5, 0.5) 'Set image source... logo.SourceFile = "C:\temp\Adventureworks.jpg" 'Set output size... logo.Width = 2 logo.LockAspectRatio = LockAspectRatio.WidthBased 'monochrome settings logo.MonochromeSettings.DitherMethod = DitherMethod.Threshold logo.MonochromeSettings.Threshold = 80 'Add items to ThermalLabel object... tLabel.Items.Add(logo) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 4, 3); tLabel.GapLength = 0.2; //Define an ImageItem object ImageItem logo = new ImageItem(0.5, 0.5); //Set image source... logo.SourceFile = @"C:\temp\Adventureworks.jpg"; //Set output size... logo.Width = 2; logo.LockAspectRatio = LockAspectRatio.WidthBased; //monochrome settings logo.MonochromeSettings.DitherMethod = DitherMethod.Threshold; logo.MonochromeSettings.Threshold = 80; //Add items to ThermalLabel object... tLabel.Items.Add(logo); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }
Example #2: In this sample, we used the same logo but rotated it 90 degrees and reversed to get a White On Black effect.
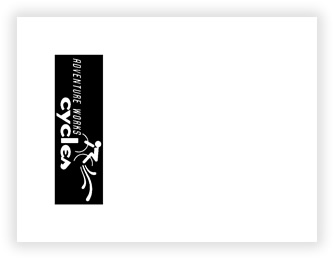
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 4, 3) tLabel.GapLength = 0.2 'Define an ImageItem object Dim logo As New ImageItem(0.5, 0.5) 'Set image source... logo.SourceFile = "C:\temp\Adventureworks.jpg" 'Set output size... logo.Width = 2 logo.LockAspectRatio = LockAspectRatio.WidthBased logo.RotationAngle = 90 'monochrome settings logo.MonochromeSettings.DitherMethod = DitherMethod.Threshold logo.MonochromeSettings.Threshold = 80 logo.MonochromeSettings.ReverseEffect = True 'Add items to ThermalLabel object... tLabel.Items.Add(logo) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 4, 3); tLabel.GapLength = 0.2; //Define an ImageItem object ImageItem logo = new ImageItem(0.5, 0.5); //Set image source... logo.SourceFile = @"C:\temp\Adventureworks.jpg"; //Set output size... logo.Width = 2; logo.LockAspectRatio = LockAspectRatio.WidthBased; logo.RotationAngle = 90; //monochrome settings logo.MonochromeSettings.DitherMethod = DitherMethod.Threshold; logo.MonochromeSettings.Threshold = 80; logo.MonochromeSettings.ReverseEffect = true; //Add items to ThermalLabel object... tLabel.Items.Add(logo); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }