In this section
Barcode Items Overview
The BarcodeItem object
A BarcodeItem – represented by Neodynamic.SDK.Printing.BarcodeItem class – lets you to generate bar codes (Linear 1D, 2D and Postal standards) that must be drawn/printed on the label. BarcodeItem objects are created by specifying some basic properties such as X, Y, Width, Height, Barcode Symbology, Value to encode, etc.
BarcodeItem objects feature the following capabilities: Data Binding, Masking and Counters, text Alignment, Rotation, barcode Sizing, rich Border settings, etc.
After creating a BarcodeItem object, it will printed on the label if you add it to the Items collection property of the ThermalLabel object.
BarcodeItem objects feature the following capabilities: Data Binding, Masking and Counters, text Alignment, Rotation, barcode Sizing, rich Border settings, etc.
After creating a BarcodeItem object, it will printed on the label if you add it to the Items collection property of the ThermalLabel object.
Working with Barcode Symbologies
In order to draw/print barcodes on a label you must specify the Symbology property to the barcode standard you want to print and the Code property to the string you want to encode into the barcode.
BarcodeItem supports most popular Linear (1D), Postal, and 2D Symbologies/Standards including Code 39, Code 128, GS1-128, GS1 DataBar (RSS-14), EAN 13 & UPC, Postal (USPS, British Royal Mail, Australia Post, DHL, etc.), Data Matrix, QR Code, PDF 417, UPS MaxiCode, Micro QR Code, ALL EAN/UPC Composite Barcodes (CC-A, CC-B & CC-C) and many more barcode standards.
Each standard/symbology requires some settings like data formatting as sizing which are exclusive to it. You can learn more about each of them by referring to the Barcode Symbology Information Center available on this help document.
BarcodeItem supports most popular Linear (1D), Postal, and 2D Symbologies/Standards including Code 39, Code 128, GS1-128, GS1 DataBar (RSS-14), EAN 13 & UPC, Postal (USPS, British Royal Mail, Australia Post, DHL, etc.), Data Matrix, QR Code, PDF 417, UPS MaxiCode, Micro QR Code, ALL EAN/UPC Composite Barcodes (CC-A, CC-B & CC-C) and many more barcode standards.
Each standard/symbology requires some settings like data formatting as sizing which are exclusive to it. You can learn more about each of them by referring to the Barcode Symbology Information Center available on this help document.
Text on barcodes
The BarcodeItem supports two kind of text to be placed along with the barcode bars:
Both kind of text do support alignment through the CodeAlignment and TextAlignment properties respectively.
- Value to encode: The most important text you find along with the barcode is called the Human Readable Text. In the BarcodeItem you specify which value to encode by using the Code property. The string set up to that property will be printed on the barcode. However, there're some situations where you may want to use other string in a different format and the like. For those cases, you can set up the HumanReadableText property. If HumanReadableText property is not empty, then it will be printed on the barcode but the barcode will always encode the string you set up to the Code property!
- Additional Text: In addition to print the text encoded into the barcode, you can provide other kind of texts for the barcode. This additional text is just informative i.e. it is not encoded into the barcode. You can specify it by using the Text property.
NOTE: 2D barcodes like Data Matrix, QR Code, PDF417, MaxiCode, etc; will not print the string you set up to the Code property. That's because these symbologies can encode non-printable characters. You can force some text to be printed along with these kind of barcodes by using either the HumandReadableText or Text properties.
Both kind of text do support alignment through the CodeAlignment and TextAlignment properties respectively.
Barcode Sizing
When you create a BarcodeItem object, you are required to specify the area (X, Y, Width & Height) where the barcode must be allocated and printed. You can size the barcode inside that area by using the following options:
- General Size: The barcode size in general depends on the value to encode (the Code property), the symbology you are encoding (the Symbology property) and dimension-related properties like BarWidth, BarRatio, BarHeight, etc. You always should specify a size (Width & Height properties) large enough to fully contain the generated barcode otherwise if the size of the generated barcode is bigger than it, then the barcode will be cut of becoming unreadable after printing.
You can also align the generated barcode inside the allocated area. This is configured by using the BarcodeAlignment property. - Fit To Size: If you want that the whole barcode (including the human readable text if any) fills the defined area, then you must set up the Sizing property to Fill option. Be careful when using this option because some barcodes can become unreadable if you force it to fill a size which is smaller than the size of the generated barcode as aforementioned stated in General Size.
Barcode Rotation
The BarcodeItem object does support rotation. The rotation can be done at any angle, in 90 degrees increments only, by specifying it to the RotationAngle property. The rotation angle range from 0 to 360 degrees measured clockwise from the x-axis.
BarcodeItem samples
The following code creates a couple of BarcodeItem objects with most of the features briefly described above.
Example #1: two linear Code 39 barcodes with borders and the human readable text with justify alignment. One of them is sizing to fill the specified area.
Example #1: two linear Code 39 barcodes with borders and the human readable text with justify alignment. One of them is sizing to fill the specified area.
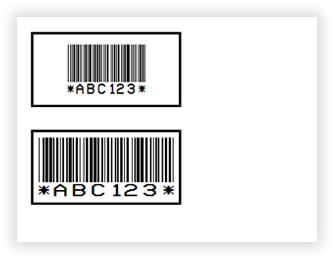
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 4, 3) tLabel.GapLength = 0.2 'Define a BarcodeItem object for encoding Code 39 symbology Dim bc1 As New BarcodeItem(0.2, 0.2, 2, 1, BarcodeSymbology.Code39, "ABC123") bc1.AddChecksum = False bc1.CodeAlignment = BarcodeTextAlignment.BelowJustify bc1.BarWidth = 0.01 bc1.BarHeight = 0.5 bc1.QuietZone = New FrameThickness(0) bc1.BarcodeAlignment = BarcodeAlignment.MiddleCenter bc1.BorderThickness = New FrameThickness(0.03) 'A second Code 39 barcode with sizing = Fill Dim bc2 As BarcodeItem = CType(bc1.Clone(), BarcodeItem) bc2.Y = 1.5 bc2.BarcodePadding = New FrameThickness(0.1) bc2.Sizing = BarcodeSizing.Fill 'Add items to ThermalLabel object... tLabel.Items.Add(bc1) tLabel.Items.Add(bc2) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 4, 3); tLabel.GapLength = 0.2; //Define a BarcodeItem object for encoding Code 39 symbology BarcodeItem bc1 = new BarcodeItem(0.2, 0.2, 2, 1, BarcodeSymbology.Code39, "ABC123"); bc1.AddChecksum = false; bc1.CodeAlignment = BarcodeTextAlignment.BelowJustify; bc1.BarWidth = 0.01; bc1.BarHeight = 0.5; bc1.QuietZone = new FrameThickness(0); bc1.BarcodeAlignment = BarcodeAlignment.MiddleCenter; bc1.BorderThickness = new FrameThickness(0.03); //A second Code 39 barcode with sizing = Fill BarcodeItem bc2 = bc1.Clone() as BarcodeItem; bc2.Y = 1.5; bc2.BarcodePadding = new FrameThickness(0.1); bc2.Sizing = BarcodeSizing.Fill; //Add items to ThermalLabel object... tLabel.Items.Add(bc1); tLabel.Items.Add(bc2); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }
Example #2: an EAN-13 barcode with supplement/add-on code and a UPC-A barcode with rounded corners and rotated 90 degrees.
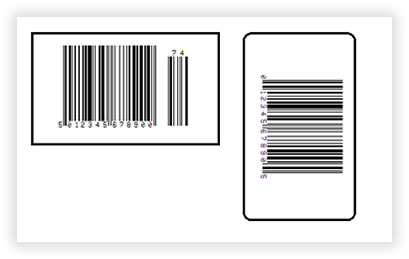
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 5, 3) tLabel.GapLength = 0.2 'Define a BarcodeItem object for encoding EAN-13 symbology Dim bc1 As New BarcodeItem(0.2, 0.2, 2.5, 1.5, BarcodeSymbology.Ean13, "5012345678900") bc1.BarWidth = 0.013 bc1.BarHeight = 1 bc1.EanUpcGuardBar = True bc1.EanUpcGuardBarHeight = bc1.BarHeight + 5 * bc1.BarWidth bc1.EanUpcSupplement = Supplement.Digits2 bc1.EanUpcSupplementCode = "74" bc1.BarcodeAlignment = BarcodeAlignment.MiddleCenter bc1.BorderThickness = New FrameThickness(0.03) bc1.Font.Size = 5 'Define a BarcodeItem object for encoding UPC-A symbology Dim bc2 As New BarcodeItem(3, 0.2, 2.5, 1.5, BarcodeSymbology.UpcA, "012345678905") bc2.BarWidth = 0.013 bc2.BarHeight = 1 bc2.EanUpcGuardBar = True bc2.EanUpcGuardBarHeight = bc1.BarHeight + 5 * bc1.BarWidth bc2.BarcodeAlignment = BarcodeAlignment.MiddleCenter bc2.BorderThickness = New FrameThickness(0.03) bc2.CornerRadius = New RectangleCornerRadius(0.1) bc2.RotationAngle = 90 bc2.Font.Size = 5 'Add items to ThermalLabel object... tLabel.Items.Add(bc1) tLabel.Items.Add(bc2) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 5, 3); tLabel.GapLength = 0.2; //Define a BarcodeItem object for encoding EAN-13 symbology BarcodeItem bc1 = new BarcodeItem(0.2, 0.2, 2.5, 1.5, BarcodeSymbology.Ean13, "5012345678900"); bc1.BarWidth = 0.013; bc1.BarHeight = 1; bc1.EanUpcGuardBar = true; bc1.EanUpcGuardBarHeight = bc1.BarHeight + 5 * bc1.BarWidth; bc1.EanUpcSupplement = Supplement.Digits2; bc1.EanUpcSupplementCode = "74"; bc1.BarcodeAlignment = BarcodeAlignment.MiddleCenter; bc1.BorderThickness = new FrameThickness(0.03); bc1.Font.Size = 5; //Define a BarcodeItem object for encoding UPC-A symbology BarcodeItem bc2 = new BarcodeItem(3, 0.2, 2.5, 1.5, BarcodeSymbology.UpcA, "012345678905"); bc2.BarWidth = 0.013; bc2.BarHeight = 1; bc2.EanUpcGuardBar = true; bc2.EanUpcGuardBarHeight = bc1.BarHeight + 5 * bc1.BarWidth; bc2.BarcodeAlignment = BarcodeAlignment.MiddleCenter; bc2.BorderThickness = new FrameThickness(0.03); bc2.CornerRadius = new RectangleCornerRadius(0.1); bc2.RotationAngle = 90; bc2.Font.Size = 5; //Add items to ThermalLabel object... tLabel.Items.Add(bc1); tLabel.Items.Add(bc2); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }
Example #3: a PDF417 barcode, a Data Matrix and a ITF-14 barcode with Bearer Bars.
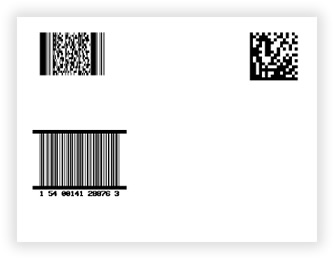
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 4, 3) tLabel.GapLength = 0.2 'Define a BarcodeItem object for encoding PDF417 symbology Dim bc1 As New BarcodeItem(0.2, 0.2, 2, 1, BarcodeSymbology.Pdf417, "PRODUCT CODE 98765") bc1.BarWidth = 0.01 bc1.BarRatio = 3 'For PDF417, bar ratio should be >= 3 'Define a BarcodeItem object for encoding DataMatrix symbology Dim bc2 As New BarcodeItem(3, 0.2, 2.5, 1.5, BarcodeSymbology.DataMatrix, "0123456789ABCDEF") bc2.DataMatrixModuleSize = 0.04 'Define a BarcodeItem object for encoding ITF-14 symbology with Bearer Bars Dim bc3 As New BarcodeItem(0.2, 1.5, 2, 1, BarcodeSymbology.Itf14, "1540014128876") bc3.BarWidth = 0.01 bc3.BarHeight = 0.7 bc3.BearerBarStyle = BearerBarStyle.HorizontalRules bc3.BearerBarThickness = 0.05 bc3.UseQuietZoneForText = True bc3.Font.Size = 5 'Add items to ThermalLabel object... tLabel.Items.Add(bc1) tLabel.Items.Add(bc2) tLabel.Items.Add(bc3) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 4, 3); tLabel.GapLength = 0.2; //Define a BarcodeItem object for encoding PDF417 symbology BarcodeItem bc1 = new BarcodeItem(0.2, 0.2, 2, 1, BarcodeSymbology.Pdf417, "PRODUCT CODE 98765"); bc1.BarWidth = 0.01; bc1.BarRatio = 3; //For PDF417, bar ratio should be >= 3 //Define a BarcodeItem object for encoding DataMatrix symbology BarcodeItem bc2 = new BarcodeItem(3, 0.2, 2.5, 1.5, BarcodeSymbology.DataMatrix, "0123456789ABCDEF"); bc2.DataMatrixModuleSize = 0.04; //Define a BarcodeItem object for encoding ITF-14 symbology with Bearer Bars BarcodeItem bc3 = new BarcodeItem(0.2, 1.5, 2, 1, BarcodeSymbology.Itf14, "1540014128876"); bc3.BarWidth = 0.01; bc3.BarHeight = 0.7; bc3.BearerBarStyle = BearerBarStyle.HorizontalRules; bc3.BearerBarThickness = 0.05; bc3.UseQuietZoneForText = true; bc3.Font.Size = 5; //Add items to ThermalLabel object... tLabel.Items.Add(bc1); tLabel.Items.Add(bc2); tLabel.Items.Add(bc3); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }