ThermalLabel SDK supports AutoShapes which are preset shapes like rectangles, circles, lines, and ellipses that can be placed on the label surface.
In This Section
Shape Items Overview
Rectangle Item
A Rectangle Item – represented by Neodynamic.SDK.Printing.RectangleShapeItem class – lets you draw rectangle shapes on the label. RectangleShapeItem objects are created by specifying some basic properties such as Width, Height, Fill, StrokeThickness, Roundness, etc.
RectangleShapeItem Sample:
By using RectangleShapeItem objects you can draw rectangles as well as rounded rectangles. The following figure is a sample label featuring rectangle shapes with different settings.
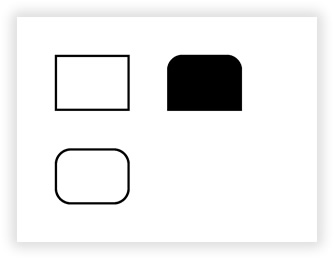
RectangleShapeItem Sample:
By using RectangleShapeItem objects you can draw rectangles as well as rounded rectangles. The following figure is a sample label featuring rectangle shapes with different settings.
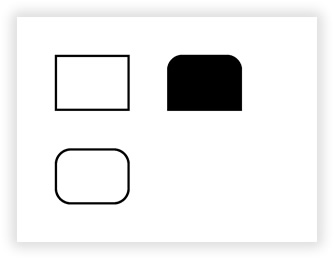
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 4, 3) tLabel.GapLength = 0.2 'Define some RectangleShapeItem objects Dim r1 As New RectangleShapeItem(0.5, 0.5, 1, 0.75) r1.StrokeThickness = 0.03 'With rounded corners Dim r2 As New RectangleShapeItem(0.5, 1.75, 1, 0.75) r2.StrokeThickness = 0.03 r2.CornerRadius = New RectangleCornerRadius(0.2) 'With some rounded corners and black fill Dim r3 As New RectangleShapeItem(2, 0.5, 1, 0.75) r3.FillColor = Neodynamic.SDK.Printing.Color.Black r3.CornerRadius = New RectangleCornerRadius(0.2, 0.2, 0, 0) 'Add items to ThermalLabel object... tLabel.Items.Add(r1) tLabel.Items.Add(r2) tLabel.Items.Add(r3) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 4, 3); tLabel.GapLength = 0.2; //Define some RectangleShapeItem objects RectangleShapeItem r1 = new RectangleShapeItem(0.5, 0.5, 1, 0.75); r1.StrokeThickness = 0.03; //With rounded corners RectangleShapeItem r2 = new RectangleShapeItem(0.5, 1.75, 1, 0.75); r2.StrokeThickness = 0.03; r2.CornerRadius = new RectangleCornerRadius(0.2); //With some rounded corners and black fill RectangleShapeItem r3 = new RectangleShapeItem(2, 0.5, 1, 0.75); r3.FillColor = Neodynamic.SDK.Printing.Color.Black; r3.CornerRadius = new RectangleCornerRadius(0.2, 0.2, 0, 0); //Add items to ThermalLabel object... tLabel.Items.Add(r1); tLabel.Items.Add(r2); tLabel.Items.Add(r3); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }
Line Item
A Line Item – represented by Neodynamic.SDK.Printing.LineShapeItem class – lets you draw lines on the label. LineShapeItem objects are created by specifying some basic properties such as Width, Height, Orientation, etc.
LineShapeItem Sample:
By using LineShapeItem objects you can draw lines at any orientation i.e. horizontal, vertical, diagonals up and down. The following figure is a sample label featuring some line shapes with different settings.
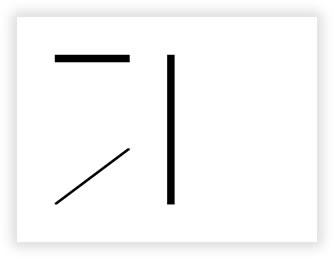
LineShapeItem Sample:
By using LineShapeItem objects you can draw lines at any orientation i.e. horizontal, vertical, diagonals up and down. The following figure is a sample label featuring some line shapes with different settings.
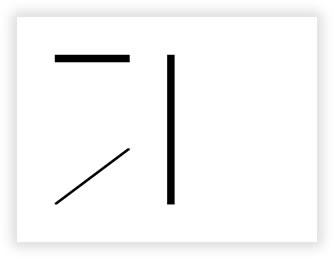
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 4, 3) tLabel.GapLength = 0.2 'Define some LineShapeItem objects 'A simple horizontal line Dim l1 As New LineShapeItem(0.5, 0.5, 1, 0.1) l1.StrokeThickness = 0.1 'A diagonal up line Dim l2 As New LineShapeItem(0.5, 1.75, 1, 0.75) l2.StrokeThickness = 0.03 l2.Orientation = LineOrientation.DiagonalUp 'A simple vertical line Dim l3 As New LineShapeItem(2, 0.5, 0.1, 2) l3.StrokeThickness = 0.1 l3.Orientation = LineOrientation.Vertical 'Add items to ThermalLabel object... tLabel.Items.Add(l1) tLabel.Items.Add(l2) tLabel.Items.Add(l3) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 4, 3); tLabel.GapLength = 0.2; //Define some LineShapeItem objects //A simple horizontal line LineShapeItem l1 = new LineShapeItem(0.5, 0.5, 1, 0.1); l1.StrokeThickness = 0.1; //A diagonal up line LineShapeItem l2 = new LineShapeItem(0.5, 1.75, 1, 0.75); l2.StrokeThickness = 0.03; l2.Orientation = LineOrientation.DiagonalUp; //A simple vertical line LineShapeItem l3 = new LineShapeItem(2, 0.5, 0.1, 2); l3.StrokeThickness = 0.1; l3.Orientation = LineOrientation.Vertical; //Add items to ThermalLabel object... tLabel.Items.Add(l1); tLabel.Items.Add(l2); tLabel.Items.Add(l3); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }
Circle and Ellipse Items
Circle and Ellipse items – represented by Neodynamic.SDK.Printing.CircleShapeItem and Neodynamic.SDK.Printing.EllipseShapeItem classes respectively– let you draw circle and ellipse/oval shapes on the label. Both kinds of objects are created by specifying some basic properties such as Width, Height, Fill, StrokeThickness, etc.
CircleShapeItem and EllipseShapeItem Sample:
By using CircleShapeItem and EllipseShapeItem objects you can draw circle, ellipse and oval shapes. The following figure is a sample label featuring such shapes.
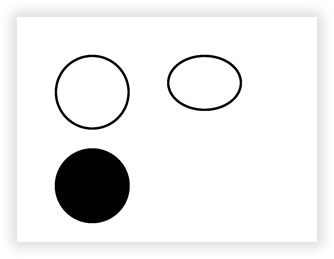
CircleShapeItem and EllipseShapeItem Sample:
By using CircleShapeItem and EllipseShapeItem objects you can draw circle, ellipse and oval shapes. The following figure is a sample label featuring such shapes.
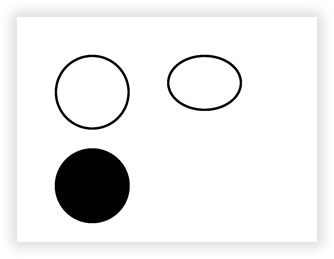
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 4, 3) tLabel.GapLength = 0.2 'Define a CircleShapeItem object Dim c1 As New CircleShapeItem(0.5, 0.5, 1) c1.StrokeThickness = 0.03 'Define a CircleShapeItem object with black fill Dim c2 As New CircleShapeItem(0.5, 1.75, 1) c2.FillColor = Neodynamic.SDK.Printing.Color.Black 'Define an EllipseShapeItem object Dim e1 As New EllipseShapeItem(2, 0.5, 1, 0.75) e1.StrokeThickness = 0.03 'Add items to ThermalLabel object... tLabel.Items.Add(c1) tLabel.Items.Add(c2) tLabel.Items.Add(e1) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 4, 3); tLabel.GapLength = 0.2; //Define a CircleShapeItem object CircleShapeItem c1 = new CircleShapeItem(0.5, 0.5, 1); c1.StrokeThickness = 0.03; //Define a CircleShapeItem object with black fill CircleShapeItem c2 = new CircleShapeItem(0.5, 1.75, 1); c2.FillColor = Neodynamic.SDK.Printing.Color.Black; //Define an EllipseShapeItem object EllipseShapeItem e1 = new EllipseShapeItem(2, 0.5, 1, 0.75); e1.StrokeThickness = 0.03; //Add items to ThermalLabel object... tLabel.Items.Add(c1); tLabel.Items.Add(c2); tLabel.Items.Add(e1); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }