In this section
ThermalLabel Overview
The ThermalLabel object
The ThermalLabel object represents the label's rectangular area intended for placing items like texts, barcodes, images, graphics, pictures, and shapes.
To design a label, the first thing to set up is the unit of measurement (inch, cm, mm, dots, point, or pica) through the UnitType property which will be used for sizing as well as items location purposes. After unit of measurement is set up, then you simply specify the label's size (Width & Height properties) and the gap separation (GapLength property) between labels on the same roll media.
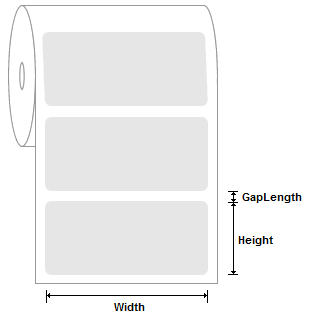
A roll media featuring single labels.
ThermalLabel object also supports multi-column label rolls i.e. the roll media contains more than one label per "row". In this case, the ThermalLabel object must be configured with the label size (Width & Height properties) of each individual label, the number of labels per row on the roll (LabelsPerRow property), the horizontal gap separation between labels (LabelsHorizontalGapLength property) and the vertical gap separation (GapLength property) between labels.
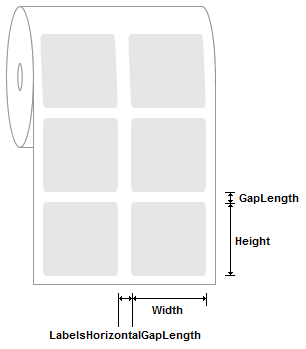
A roll media featuring 2 labels per row i.e. a multi-column label layout.
You can create as many items as you want to print on the label. Items are simple objects which are assembled together to design and create the output thermal label. All items are printed in the order that each of them has in the stack of items (which is the Items property of the ThemalLabel object) and in the location specified for each item through their X and Y properties. There are four main kinds of items which can be placed on the label: TextItem, BarcodeItem, ImageItem, and ShapeItem (Lines, Rectangles, Circles, etc.)
Finaly, the PrintJob is the object that specifies information about how the ThermalLabel object is printed, including the printer device settings and communication, label orientation, number of copies, etc. It also is used for exporting or saving a ThermalLabel object to raster image formats or Adobe PDF documents which is very useful when you are in development/test phase or have no access to a physical thermal printer.
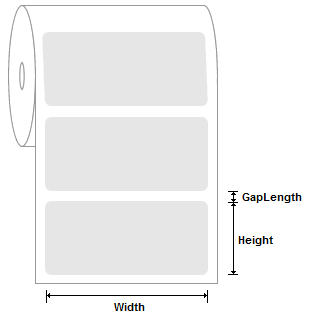
A roll media featuring single labels.
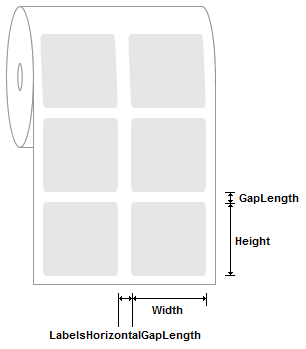
A roll media featuring 2 labels per row i.e. a multi-column label layout.
Finaly, the PrintJob is the object that specifies information about how the ThermalLabel object is printed, including the printer device settings and communication, label orientation, number of copies, etc. It also is used for exporting or saving a ThermalLabel object to raster image formats or Adobe PDF documents which is very useful when you are in development/test phase or have no access to a physical thermal printer.
Designing a Basic Label
In this section you'll learn how to design a simple label with a text and a barcode items. In this sample, the physical label size is 4 inch wide and 3 inch high. On that label, we'll place a TextItem displaying "Thermal Label Test" string and a BarcodeItem encoding the "ABC 12345" string in Code 128 Symbology. The label to design will look like the following:
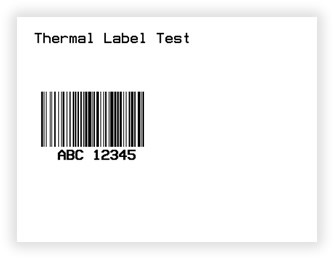
The following is the VB.NET and C# codes for generating that basic label. You should create a .NET project like a Windows Forms App and use the code below to print the label. You must have a Thermal Printer connected to your machine and a Windows Driver installed on your system or you can save the the label output to a raster image format or Adobe PDF. Notice that the printing process is launched by a PrintJob object.
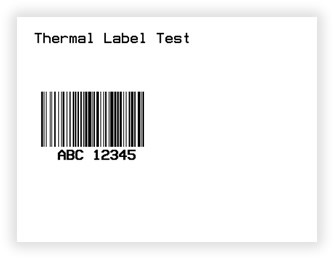
The following is the VB.NET and C# codes for generating that basic label. You should create a .NET project like a Windows Forms App and use the code below to print the label. You must have a Thermal Printer connected to your machine and a Windows Driver installed on your system or you can save the the label output to a raster image format or Adobe PDF. Notice that the printing process is launched by a PrintJob object.
Visual Basic
'Define a ThermalLabel object and set unit to inch and label size Dim tLabel As New ThermalLabel(UnitType.Inch, 4, 3) tLabel.GapLength = 0.2 'Define a TextItem object Dim txtItem As New TextItem(0.2, 0.2, 2.5, 0.5, "Thermal Label Test") 'Define a BarcodeItem object Dim bcItem As New BarcodeItem(0.2, 1, 2, 1, BarcodeSymbology.Code128, "ABC 12345") 'Set bars height to .75inch bcItem.BarHeight = 0.75 'Set bars width to 0.0104inch bcItem.BarWidth = 0.0104 'Add items to ThermalLabel object... tLabel.Items.Add(txtItem) tLabel.Items.Add(bcItem) 'Create a PrintJob object Using pj As New PrintJob() 'Create PrinterSettings object Dim myPrinter As New PrinterSettings() myPrinter.Communication.CommunicationType = CommunicationType.USB myPrinter.Dpi = 203 myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL myPrinter.PrinterName = "Zebra TLP2844-Z" 'Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter 'Print ThermalLabel object... pj.Print(tLabel) End Using
C#
//Define a ThermalLabel object and set unit to inch and label size ThermalLabel tLabel = new ThermalLabel(UnitType.Inch, 4, 3); tLabel.GapLength = 0.2; //Define a TextItem object TextItem txtItem = new TextItem(0.2, 0.2, 2.5, 0.5, "Thermal Label Test"); //Define a BarcodeItem object BarcodeItem bcItem = new BarcodeItem(0.2, 1, 2, 1, BarcodeSymbology.Code128, "ABC 12345"); //Set bars height to .75inch bcItem.BarHeight = 0.75; //Set bars width to 0.0104inch bcItem.BarWidth = 0.0104; //Add items to ThermalLabel object... tLabel.Items.Add(txtItem); tLabel.Items.Add(bcItem); //Create a PrintJob object using (PrintJob pj = new PrintJob()) { //Create PrinterSettings object PrinterSettings myPrinter = new PrinterSettings(); myPrinter.Communication.CommunicationType = CommunicationType.USB; myPrinter.Dpi = 203; myPrinter.ProgrammingLanguage = ProgrammingLanguage.ZPL; myPrinter.PrinterName = "Zebra TLP2844-Z"; //Set PrinterSettings to PrintJob pj.PrinterSettings = myPrinter; //Print ThermalLabel object... pj.Print(tLabel); }